After a loved one passes away, your mind and heart go into a state of panic. First there’s the disbelief that you won’t ever see them again. You have recurrences of seeing them out of the corner of your eye. Hearing them only to discover it was someone or something else. Each time this happens, your mind remembers that they’re gone and your heart breaks all over again. As you work through it, you become obsessed with remembering the mundane things about them: the sound of their voice, things they’d say at certain parts of the day, little looks they’d give you. This obsession is fueled by the fact that you know you will forget many of these things, and you don’t want to. You know that your mind is built to forget, and by it’s nature you will end up not caring as intensely as you do at that moment. It is a survival mechanism, it is unavoidable, it is heart-breaking. You can’t shut down the pain, but you can make yourself busy to think less of how much you are hurt. I spent my full free time this period on my second hobby – poker, where I played hundreds and hundreds of hours.
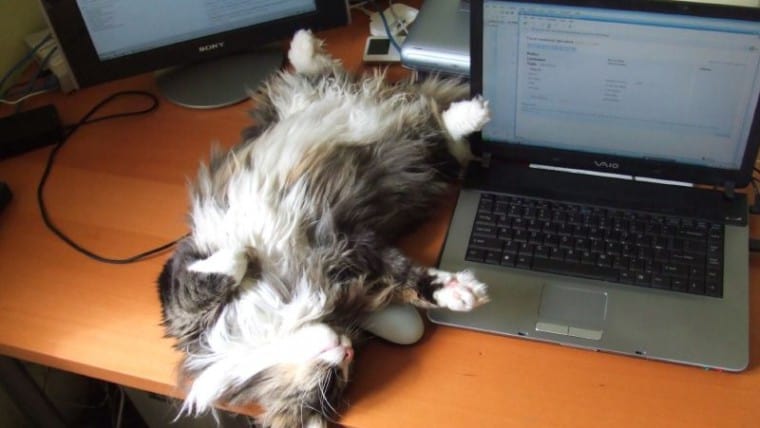